Algorithms
Data Structures & Algorithms evaluate a candidate's problem-solving skills, coding proficiency, and understanding of computer science fundamentals. These questions often cover data structures, algorithms, system design, and programming concepts.
Subsets Or Powerset
In subsets or powerset problem, we need to write a program that finds all possible subsets (the power set) of a given input. The solution set must not contain duplicate elements.

Challenge 2: Check If a Given Number is Even/Odd
This is an example of another Bitwise question. Think of the rightmost bit of a number and think of some logic that could solve this.
Counting Bits II
This is an extension to the counting set bits problem, counting the number of set bits or 1's present in a number. In here we see solutions in Java, JavaScript, Cpp, and TypeScript.
Challenge 1: Count Set Bits Or Number of 1 bit's
This is a traditional problem counting the number of set bits or 1's present in a number. Let’s see how we can count the set bits using the AND operator.
Convert Decimal Numbers to Binary Numbers
We use the modulo and division method, and we calculate the binary representation of a decimal number. Let’s represent the binary by considering the remainder column from bottom to top.
Count the Number of Digits in an Integer
This is an example problem for counting digits in an integer. Solving this problem helps you find the place values and how they are represented in the decimal number system. Let’s see some approaches we can take to solve this algorithmic problem. Approach
Leetcode 896: Monotonic Array
An array is called monotonic if the index of each element increases from the first to the last or decreases from the first to the last.
Find The Missing Number From The Array
In this article, we'll learn various ways to find the missing number in an array. We use a memoization approach, a mathematical approach, and the most optimized way using the XOR operator.
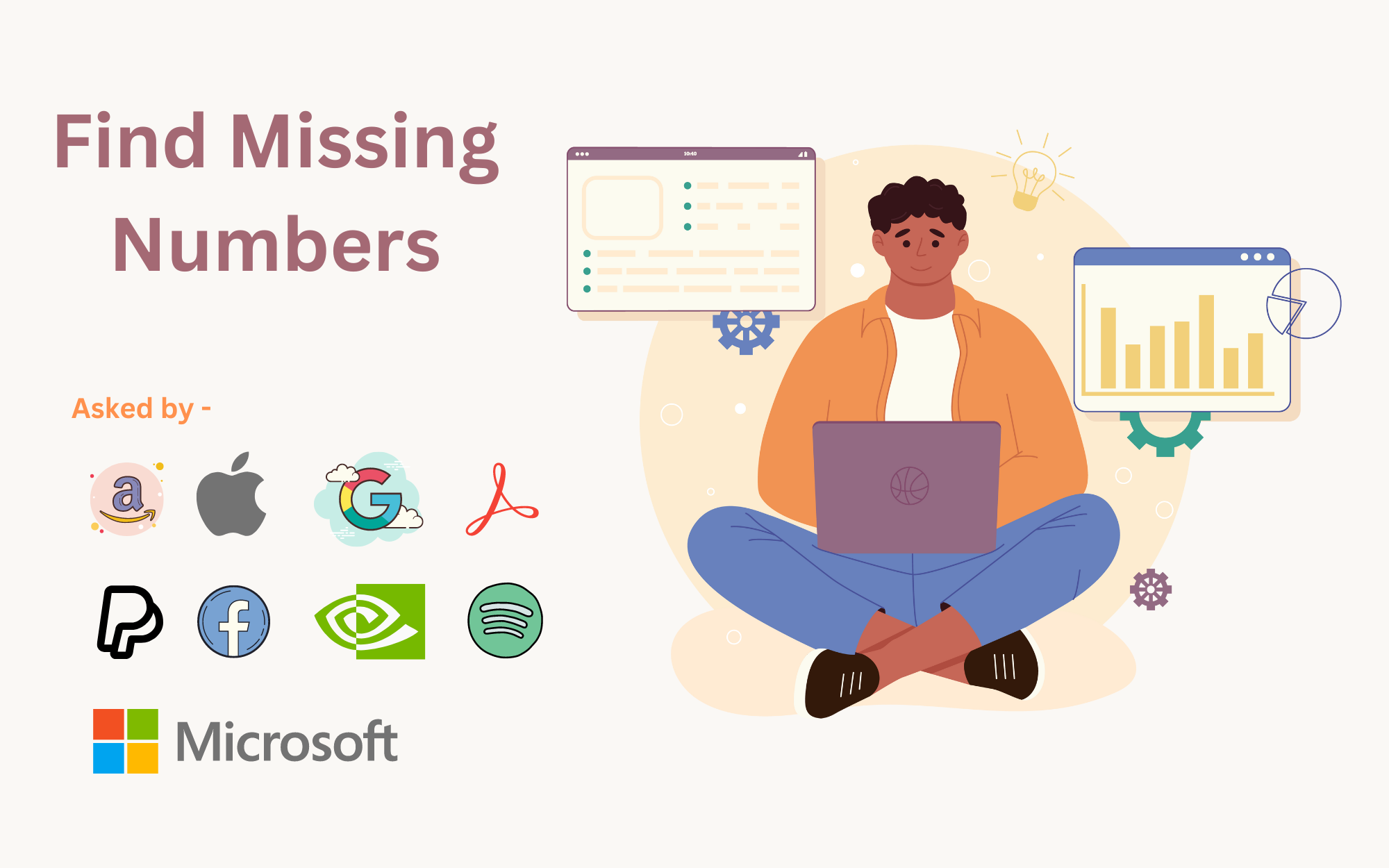